C# - 面向对象 - 多态
虚方法与方法重写
方法重写override
- 修改基类的某些方法的执行逻辑。
- 使用override关键词。
重写的作用 —— 实现多态
实例
Canvas.cs
namespace HelloWord
{
class Canvas
{
public void DrawShapes(List<Shape> shapes)
{
foreach (var shape in shapes)
{
shape.Draw();
}
}
}
}
Position.cs
namespace HelloWord
{
public class Position
{
public int X { get; set; }
public int Y { get; set; }
}
}
Program.cs
using System;
using System.Collections;
using System.Collections.Generic;
namespace HelloWord
{
class Program
{
static void Main(string[] args)
{
var shapeList = new List<Shape>();
shapeList.Add(new Circle());
shapeList.Add(new Rectange());
var canvas = new Canvas();
canvas.DrawShapes(shapeList);
Console.Read();
}
}
}
Shape.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace HelloWord
{
public class Circle:Shape {
public override void Draw()
{
Console.WriteLine("画圆");
}
}
public class Rectange : Shape
{
public override void Draw()
{
Console.WriteLine("画方");
}
}
public class Shape
{
public int Width { get; set; }
public int Height { get; set; }
public Position Position { get; set; }
public virtual void Draw() {
Console.WriteLine("绘制图案");
}
}
}
ShapeType.cs
namespace HelloWord
{
public enum ShapeType
{
Circle,
Rectangle,
trangle
}
}
什么是多态
多态的好处
抽象类与抽象成员
abstract关键词
- 声明修饰符
- 可以修饰类、方法、属性。
- 只有声明、没有逻辑,不会被实现。
抽象语法
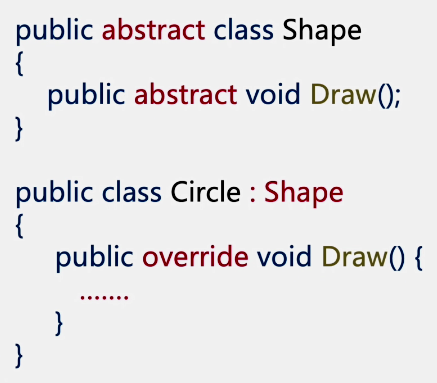
抽象规则
- 声明为abstract以后,这个属性或者方法是不可以有代码实现。
- 当某个成员被声明为abstract,整个class都需要被声明为抽象类。
- 派生类必须实现抽象类中所声明的所有抽象方法和抽象属性。
- 抽象类不可以实例化。
为什么要使用抽象
- 只是一种概念、一种设计方式的集中体现。
- 规范代码的开发设计思路。
- 从业务层面上避免错误出现。
密封类(sealed类)和密封成员(sealed成员)
- 使用sealed修饰符。
- 防止类继承、防止派生类重写。
- sealed修饰符不仅可用来修饰class,同样也可以修饰类成员。
例子
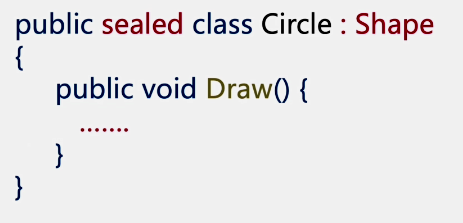
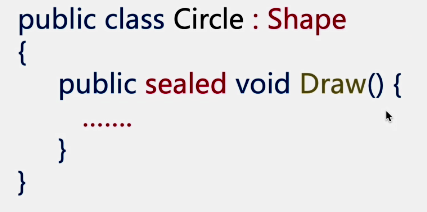
为什么要使用sealed
- 运行效率上会有一点点的提升。
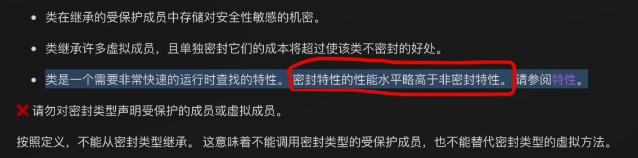
可以使用sealed的场景
- 静态类。
- 需要存储敏感的数据。
- 虚方法太多,重写的代价过高的时候。
- 追求性能提升。
- C#中的字符串类,
system.string
,密封的,不可继承。 - 可以使用
extension
做方法拓展。
Comments | NOTHING