C# - 基础语法 - C#编程上手指南
C#编程上手指南
Console.Read();
- Console.Read()方法用于从标准输入流中读取下一个字符。
- 为了不让执行main函数后的方法时退出。
namespace helloworld
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("hello world2");
Console.Read();
}
}
}
System.Console
- IO操作工具集(i代表input,o代表output)。
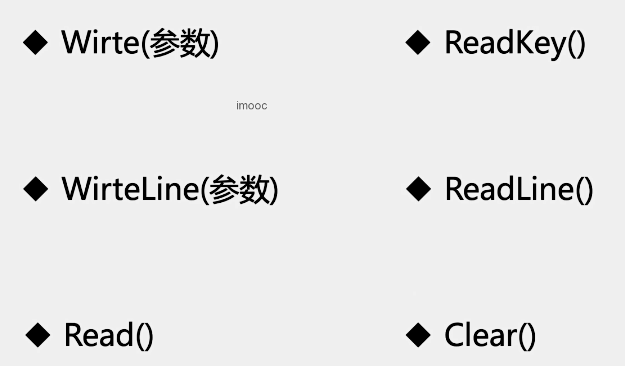
Wirte & WirteLine
namespace helloworld
{
internal class Program
{
static void Main(string[] args)
{
//WriteLine()、自动换行
Console.WriteLine("WriteLine()输出效果");
Console.WriteLine("你");
Console.WriteLine("好");
Console.WriteLine("世界");
Console.WriteLine("------------------------");
//write()、不会自动换行、合并在一块
Console.WriteLine("write()输出效果");
Console.Write("你");
Console.Write("好");
Console.Write("世界");
//Console.Read()方法用于从标准输入流中读取下一个字符。
Console.Read();
}
}
}
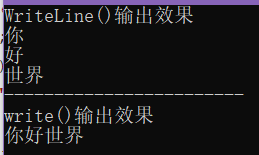
Read(),ReadKey(),以及 ReadLine()
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace helloworld
{
internal class Program
{
static void Main(string[] args)
{
//输入A,输出65
//数字
//Console.Read()方法用于从标准输入流中读取下一个字符
int a = Console.Read();
Console.WriteLine(a);
//字符串,读取一行
//ReadLine:读取下一行字符,在窗口停留一下,直到用户按下回车键之后关闭命令窗口,一次读取一行字符:
String b = Console.ReadLine();
Console.WriteLine(b);
//ReadKey():获取用户按下的下一个字符或者功能键,
//这个函数是为了在控制台窗口停留一下,直到敲击键盘为止,就是用户按下任意键后就会退出命令窗口,一次读入一个字符;
ConsoleKeyInfo c = Console.ReadKey();
Console.WriteLine(c.Key);
//console.clear() 方法用于清除控制台所有信息
Console.Clear();
Console.Read();
}
}
}
变量(Variable)与数据类型(Data Type)
变量(Variable)
变量的基本定义
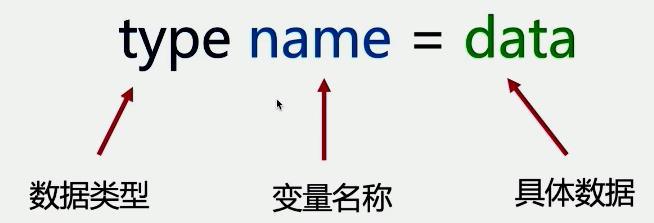
C#中的重要类型
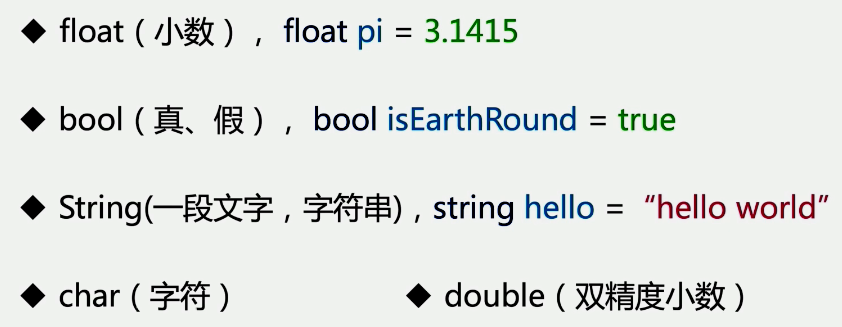
例
namespace variable3
{
internal class Program
{
static void Main(string[] args)
{
int number1;
number1 = 13;
Console.WriteLine(number1);
int number2, number3, number4;
Console.Read();
}
}
}
数据类型(Data Type)
基本数据类型
- C#有13种基本数据类型
- 预先定义好的内建类型,可以直接使用
- Primitive Types
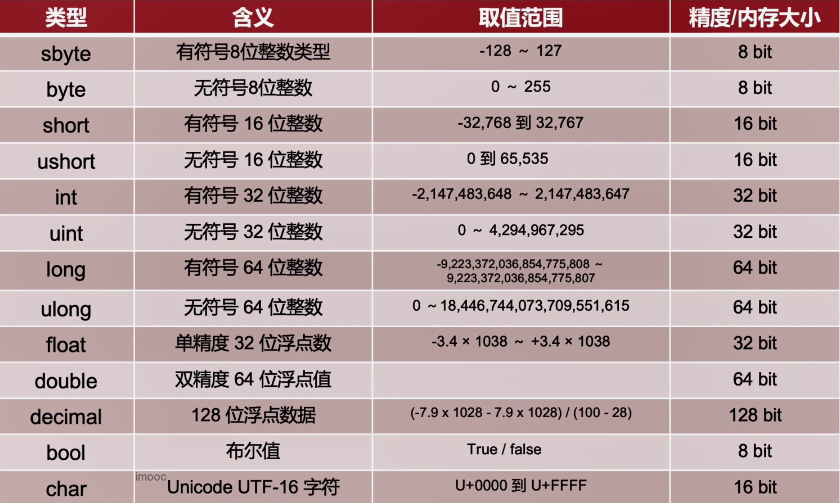
byte 与 sbyte
short 与 ushort
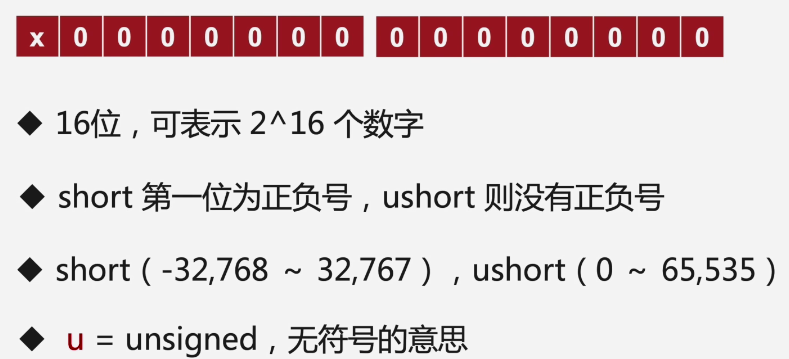
int 与 uint
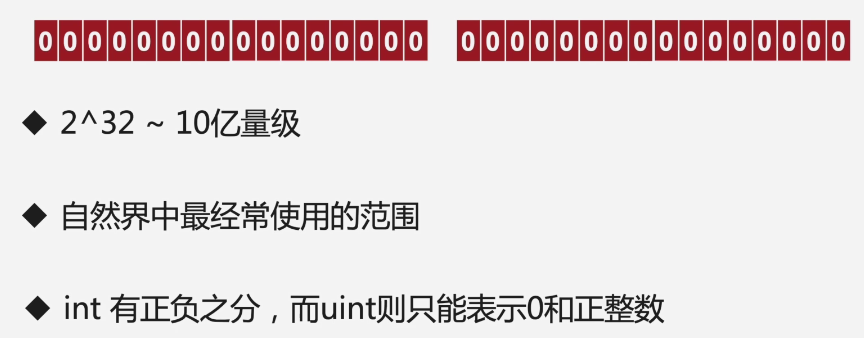
long 与 ulong
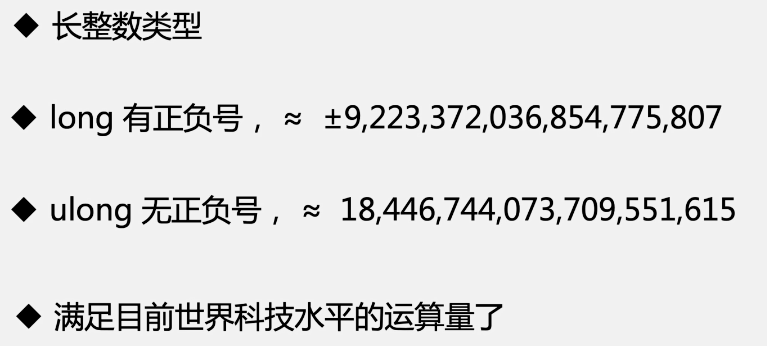
小数
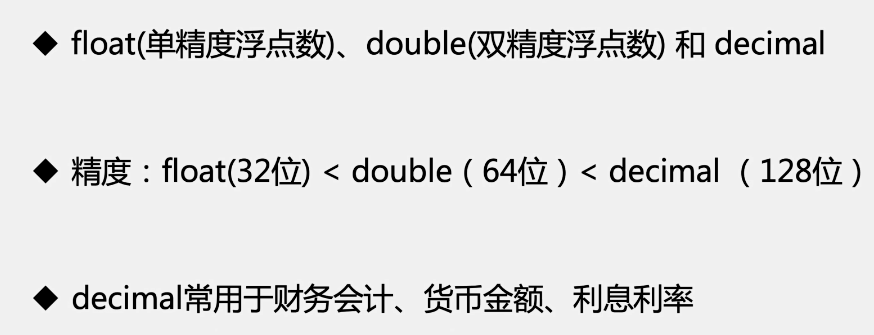
bool 与 char
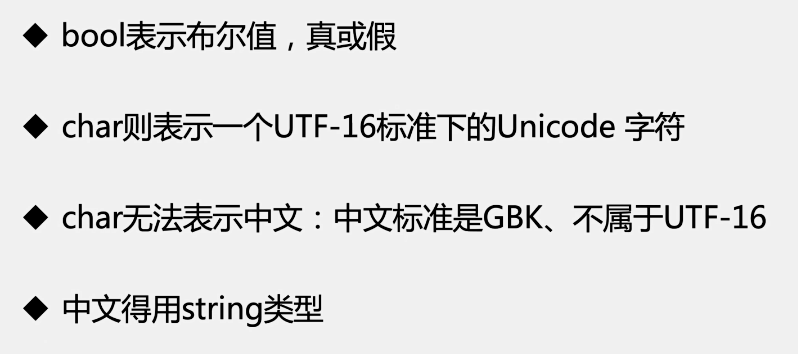
内建类型,但不属于基本类型
- 中文得用string类型,string可以通过把多个Unicode字符拼接起来,显示中文、甚至是emoji。
- object对象类型
- dynamic动态类型
字符串方法与操作
namespace @string
{
internal class Program
{
static void Main(string[] args)
{
string hello = "hello world";
String hello2 = "hello world";
//字符串拼接
Console.WriteLine(hello + "li");
string message = hello + "li";
//字符串格式化处理
string name = "li";
int age = 18;
Console.WriteLine("my name is {0}, i am {1} years old", name, age);
string message2 = "my name is {0}, i am {1} years old";
string output = string.Format(message2, name, age);
Console.WriteLine(output);
//字符串内嵌
string message3 = $"my name is {name}, i am {age} years old";
Console.WriteLine(message3);
//原意字符串
string message4 = @"my name is {name},\n
i am {age} years old.\n
i am a devloper";
Console.WriteLine(message4);
//转大写字符
string h = hello.ToUpper();
Console.WriteLine(h);
Console.Read();
}
}
}
决策与分支
代码决策 decisions
if
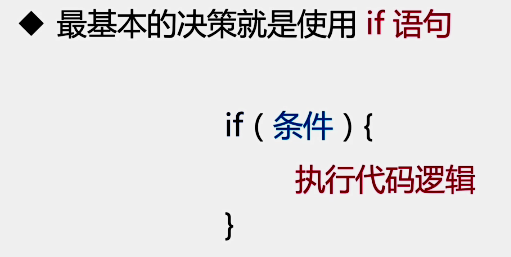
switch
- switch
- case
- break ( return )
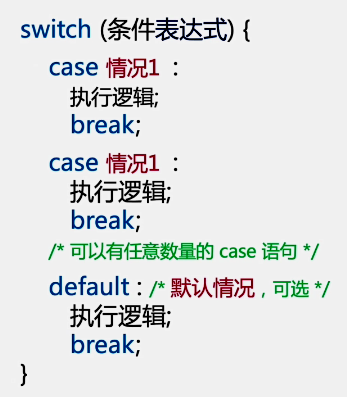
?∶操作符(三元操作)
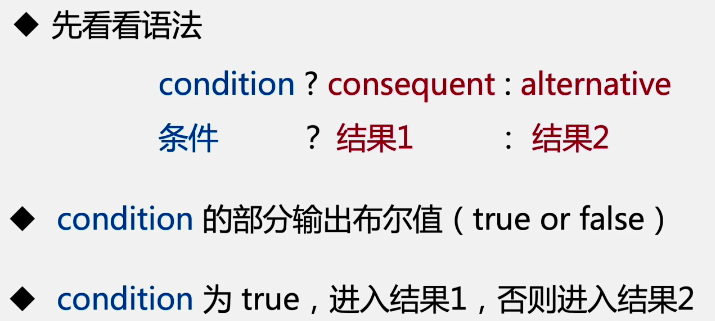
客户管理系统(控制台应用程序)
实例(if、else、switch)
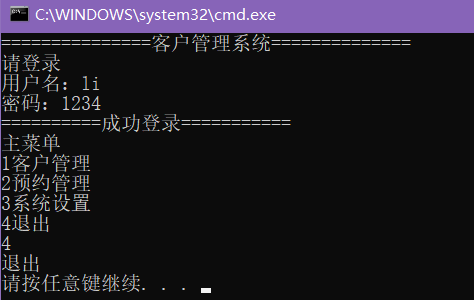
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CMS
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("===============客户管理系统==============");
Console.WriteLine("请登录");
string password;
string username;
Console.Write("用户名:");
username = Console.ReadLine();
if (username == "li")
{
Console.Write("密码:");
password = Console.ReadLine();
if (password == "1234")
{
Console.WriteLine("==========成功登录===========");
Console.WriteLine("主菜单");
Console.WriteLine("1客户管理");
Console.WriteLine("2预约管理");
Console.WriteLine("3系统设置");
Console.WriteLine("4退出");
string selection = Console.ReadLine();
switch (selection) {
case "1":
Console.WriteLine("1客户管理");
break;
case "2":
Console.WriteLine("1预约管理");
break;
case "3":
Console.WriteLine("1系统设置");
break;
case "4":
default:
Console.WriteLine("退出");
break;
}
}
else {
Console.WriteLine("密码错误");
}
}
else {
Console.WriteLine("没有这个用户");
}
}
}
}
程序循环
- 节省程序员大量的开发精力
- 轻易的重复某段代码或者某个功能模块
- 可以处理大量数据
循环类型
for循环
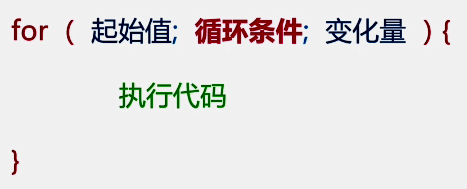
实例
namespace _05loop
{
internal class Program
{
static void Main(string[] args)
{
for (int i = 0; i < 10; i++) {
Console.WriteLine("hello loop");
}
Console.Read();
}
}
}
while循环
- 当...的时候
- 先进行条件检查,只有当条件满足的时候才进入循环。
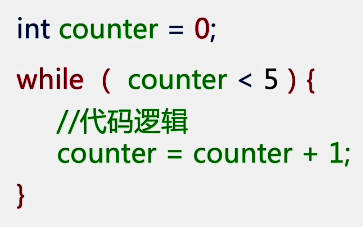
实例
namespace _05loop
{
internal class Program
{
static void Main(string[] args)
{
//while循环
int j = 0;
while (j < 5) {
Console.WriteLine("hello loop while");
//如果没有++,循环不停止
j++;
}
Console.ReadLine();
}
}
}
do while循环
- while循环的亲戚。
- 先循环、再检查。
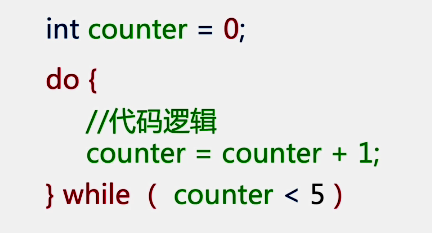
实例
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _05loop
{
internal class Program
{
static void Main(string[] args)
{
//do while循环
int k = 0;
do
{
Console.WriteLine("hello loop do while");
//如果没有++,循环不停止
k++;
} while (k < 5);
Console.ReadLine();
}
}
}
循环终止 break
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _05loop
{
internal class Program
{
static void Main(string[] args)
{
//for循环
for (int i = 0; i < 10; i++) {
Console.WriteLine("hello loop");
if (i == 3) {
Console.WriteLine("循环终止");
break;
}
}
}
}
}
只跳过本次循环,continue
namespace _05loop
{
internal class Program
{
static void Main(string[] args)
{
for (int i = 0; i < 10; i++)
{
if (i == 3)
{
Console.WriteLine("循环终止");
//只跳过本次循环,continue
continue;
}
Console.WriteLine("hello loop continue");
}
Console.ReadLine();
}
}
}
跳过所有偶数
namespace _05loop
{
internal class Program
{
static void Main(string[] args)
{
for (int i = 0; i < 10; i++)
{
//跳过偶数 i%2 == 0
if (i%2 == 0)
{
Console.WriteLine("跳过偶数");
//break;
//只跳过本次循环,continue
continue;
}
Console.WriteLine("hello loop 跳过偶数");
}
Console.ReadLine();
}
}
}
客户管理系统(控制台应用程序)—— 循环
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CMS
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("===============客户管理系统==============");
Console.WriteLine("请登录");
bool isExit = false;
do {
string password;
string username;
Console.Write("用户名:");
username = Console.ReadLine();
if (username != "li")
{
Console.WriteLine("查无此人");
continue;
}
Console.Write("密码:");
password = Console.ReadLine();
if (password != "1234")
{
Console.WriteLine("密码错误");
continue;
}
while (!isExit) {
Console.WriteLine("==========成功登录===========");
Console.WriteLine("主菜单");
Console.WriteLine("1客户管理");
Console.WriteLine("2预约管理");
Console.WriteLine("3系统设置");
Console.WriteLine("4退出");
string selection = Console.ReadLine();
switch (selection) {
case "1":
Console.WriteLine("1客户管理");
break;
case "2":
Console.WriteLine("2预约管理");
break;
case "3":
Console.WriteLine("3系统设置");
break;
case "4":
default:
Console.WriteLine("退出");
isExit = true;
break;
}
}
} while (!isExit);
Console.Read();
}
}
}
方法
- 花括号包起来的代码块
- 可以调用
- 可以执行
- 在C#中,所有的指令都必须在方法中才能执行。
方法签名
访问修饰符
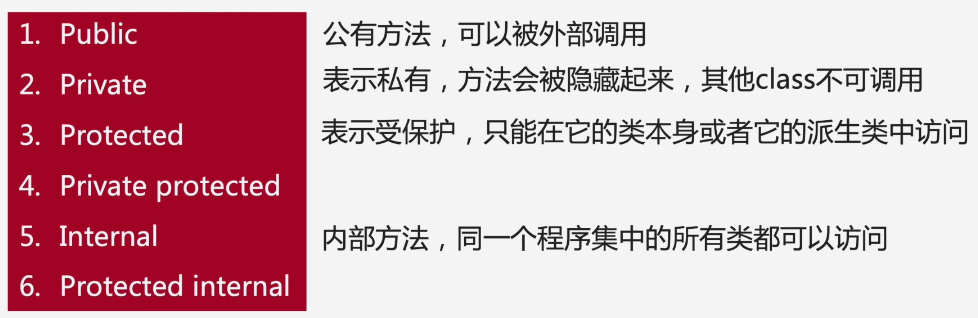
声明修饰符
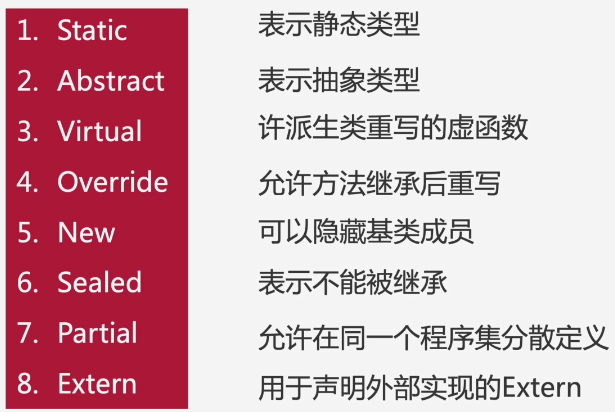
实例
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _07Method_signature
{
internal class Program
{
//找最大数
public static int MaxNum(int num1, int num2)
{
int result;
if (num1 > num2)
{
result = num1;
}
else
{
result = num2;
}
return result;
}
static void Main(string[] args)
{
int output = MaxNum(1, 99);
Console.WriteLine(output);
Console.Read();
}
}
}
方法签名-总结
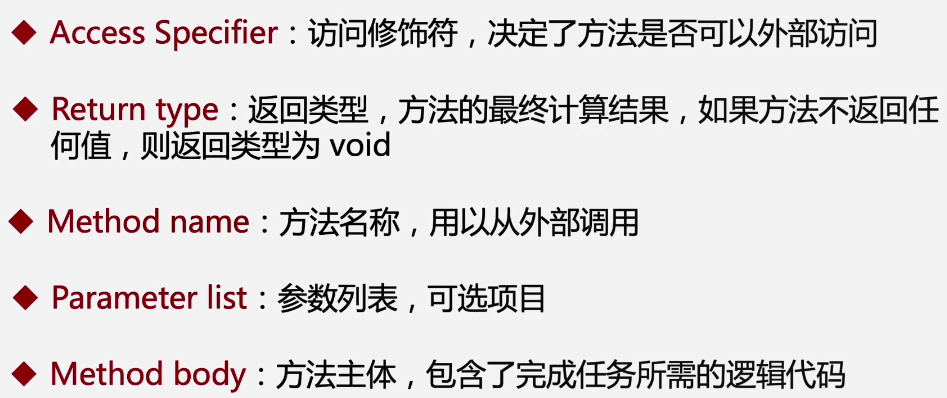
形参与实参
- 形参,parameter,形式上的参数。
- 实参,argument,真正调用方法过程中传入的具体数据。
形参
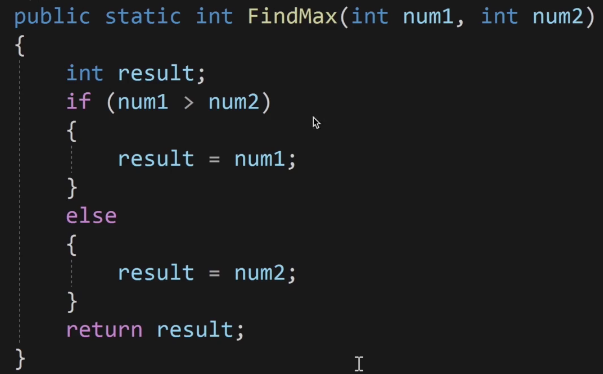
实参

判断形参与实参
方法返回
- return,方法的返回值,也就是方法的最终计算结果。
- 如果方法不返回任何值,则返回类型为void。
- 使用return则意味着方法彻底停止。
- 特殊需要,你也可以使用return来提前结束代码。
方法表达式 =>
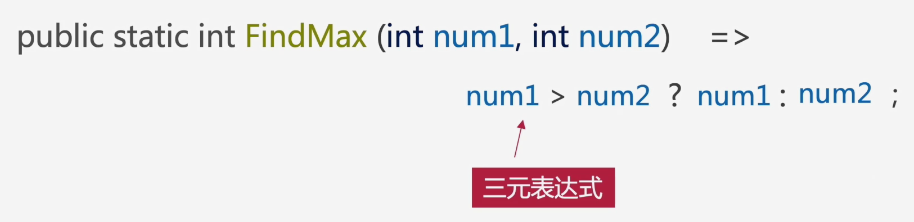
函数化用户登录过程 —— 客户管理系统(控制台应用程序)
namespace CMS
{
internal class Program
{
static string CmdReader(string ins)
{
Console.WriteLine(ins);
string cmd = Console.ReadLine();
return cmd;
}
static void Main(string[] args)
{
Console.WriteLine("===============客户管理系统==============");
Console.WriteLine("请登录");
bool isExit = false;
do {
string password;
string username;
/*Console.Write("用户名:");
username = Console.ReadLine();*/
username = CmdReader("用户名:");
if (username != "li")
{
Console.WriteLine("查无此人");
continue;
}
/* Console.Write("密码:");
password = Console.ReadLine();*/
password = CmdReader("密码:");
if (password != "1234")
{
Console.WriteLine("密码错误");
continue;
}
while (!isExit) {
/* Console.WriteLine("==========成功登录===========");
Console.WriteLine("主菜单");
Console.WriteLine("1客户管理");
Console.WriteLine("2预约管理");
Console.WriteLine("3系统设置");
Console.WriteLine("4退出");
string selection = Console.ReadLine();*/
string selection = CmdReader("主菜单\n1客户管理\n2预约管理\n3系统设置\n4退出\n");
switch (selection) {
case "1":
Console.WriteLine("1客户管理");
break;
case "2":
Console.WriteLine("2预约管理");
break;
case "3":
Console.WriteLine("3系统设置");
break;
case "4":
default:
Console.WriteLine("退出");
isExit = true;
break;
}
}
} while (!isExit);
Console.Read();
}
}
}
值传参、引用传参、输出传参
值传参
- 参数传递的默认方式。
- 为每个值参数创建一个新的存储位置,实际参数的值会复制给形参,实参和形参使用的是两个不同内存中的值。
- 当形参的值发生改变时,不会影响实参的值,实参数据安全。
引用传参
namespace _09Pass_by_value
{
internal class Program
{
//ref
//引用传参
static void swap(ref int x, ref int y) {
int temp;
temp = x;
x= y;
y= temp;
}
static void Main(string[] args)
{
int a = 1;
int b = 2;
//ref
swap(ref a, ref b);
Console.WriteLine("A" + a);
Console.WriteLine("B" + b);
Console.ReadLine();
}
}
}
输出传参
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _09Pass_by_value
{
internal class Program
{
//输出传参
static void getValue(out int x) {
x = 6;
}
static void Main(string[] args)
{
int a = 1;
int b = 2;
getValue(out a);
Console.WriteLine("第二个A" + a);
Console.ReadLine();
}
}
}
Comments | NOTHING