C# - 面向对象 - 入门
面向对象
- 原则:使用对象来描述世界中客观存在的一切事物。
- 世界的运行过程就是不同对象互相作用的结果。
- 面向对象要求我们用代码来模拟真实世界的客观运行规律。
- 对象、类、实例
- 从宏观上来认识、分解并且总结某个事物的运行规则。
对象
- C#中,一切皆是对象。
- 类是对象、实例是对象、变量也是对象,甚至数据类型也是对象。
- 能被执行的动作,是对象的调用方法。
- 而可以被量化的描述,则是对象的基本属性。
类Class
实例instance
- 类表示一类事物。
- 实例表示这类事物中的某一个具体的个体。
类、对象、与 实例 的关系
- 每个对象都是某个类(class)的一个实例。
- 对象与实例可以划上约等号,对象 ≈ 实例。
- 创建一个对象的过程就叫做实例化,而实例化的产物就叫做实例、或者叫做对象。
面向对象的意义
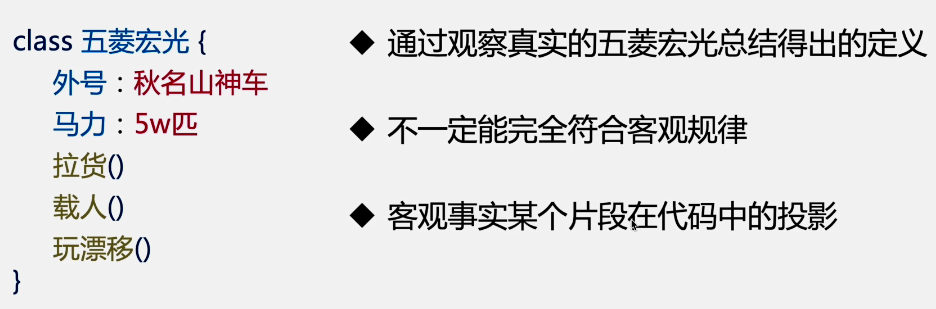
客户管理系统 —— 用户管理 —— 面向对象
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CMS
{
internal class Program
{
static string CmdReader(string ins)
{
Console.WriteLine(ins);
string cmd = Console.ReadLine();
return cmd;
}
public class User {
public bool isUserLogin;
public void Login() {
string password;
string username;
username = CmdReader("用户名:");
if (username != "li")
{
Console.WriteLine("查无此人");
return;
}
password = CmdReader("密码:");
if (password != "1234")
{
Console.WriteLine("密码错误");
return;
}
isUserLogin = true;
}
}
public class Menu {
public void ShowMenu() {
bool isExit = false;
while (!isExit)
{
string selection = CmdReader("主菜单\n1客户管理\n2预约管理\n3系统设置\n4退出\n");
switch (selection)
{
case "1":
Console.WriteLine("1客户管理");
break;
case "2":
Console.WriteLine("2预约管理");
break;
case "3":
Console.WriteLine("3系统设置");
break;
case "4":
default:
Console.WriteLine("退出");
isExit = true;
break;
}
}
}
}
public class CMSController {
public void Start(User user,Menu menu) {
//login
do
{
user.Login();
} while (!user.isUserLogin);
//显示菜单
menu.ShowMenu();
}
}
static void Main(string[] args)
{
//初始化用户系统
User user = new User();
//初始化菜单系统
Menu menu = new Menu();
//初始化CMSController
CMSController controller = new CMSController();
Console.WriteLine("===============客户管理系统==============");
Console.WriteLine("请登录");
controller.Start(user,menu);
Console.Read();
}
}
}
类class与实例instance
实例:输出坐标点数据
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
//输出坐标点数据
namespace _11classANDinstance
{
internal class Program
{
static void Main(string[] args)
{
//x:15,y:10
Point a = new Point();
a.x= 1;
a.y= 2;
DrawPoint(a);
Console.Read();
return;
}
public class Point {
public int x, y;
}
public static void DrawPoint(Point point) =>
Console.WriteLine($"x为:{point.x},y: {point.y}");
}
}
对象聚合Cohesion
高内聚,低耦合
//对象聚合Cohesion
//高内聚,低耦合
namespace _12Cohesion
{
internal class Program
{
static void Main(string[] args)
{
//x:15,y:10
Point a = new Point();
a.x= 1;
a.y= 2;
a.DrawPoint();
Point b = new Point();
b.x= 5;
b.y= 6;
double resule = a.GetDistances(b);
Console.WriteLine(resule);
Console.Read();
return;
}
public class Point {
public int x, y;
public void DrawPoint() =>
Console.WriteLine($"x为:{x},y: {y}");
public double GetDistances(Point p) =>
Math.Pow(x - p.x, 2) + Math.Pow(y - p.y, 2);
}
}
}
构造方法与方法重载
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
//构造方法与方法重载
namespace _13ConstructedMethodsAndMethodOverloading
{
internal class Program
{
static void Main(string[] args)
{
//x:15,y:10
Point a = new Point(15,10);
a.DrawPoint();
Point b = new Point(22,120);
double resule = a.GetDistances(b);
Console.WriteLine(resule);
Console.Read();
return;
}
public class Point {
public int x, y;
public Point()
{
this.x = 15;
y = 10;
}
public Point(int x,int y) {
this.x = 15;
this.y = 10;
}
public Point(int x)
{
this.x = x;
this.y = 10;
}
public void DrawPoint() =>
Console.WriteLine($"左边点为 x: {x}, y: {y}");
public double GetDistances(Point p) =>
Math.Pow(x - p.x, 2) + Math.Pow(y - p.y, 2);
}
}
}
访问修饰符
- public
- private
- protected
- internal
字段、属性与对象封装
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
//字段、属性与对象封装
namespace _15FieldPropertyAndObjectEncapsulation
{
internal class Program
{
static void Main(string[] args)
{
//x:15,y:10
Point a = new Point(15,10);
a.DrawPoint();
Point b = new Point(22,120);
double resule = a.GetDistances(b);
Console.WriteLine(resule);
// public, private, protected, internal
Point point = new Point(25, 30);
point.X = 30;
Console.WriteLine(point.X);
Console.Read();
return;
}
public class Point {
// 成员变量x轴,使用属性
private int _x;
public int X {
get { return this._x; }
set {
if(value < 0)
{
throw new Exception("value不能小于0");
}
this._x = value;
}
}
// 成员变量y轴,使用setY getY方法
private int y;
public void SetY(int value)
{
if (value < 0)
{
throw new Exception("value不能小于0");
}
this.y = value;
}
public int GetY() => this.y;
// 成员变量z轴,自动生成getter、setter
public int Z { get; set; }
public Point()
{
this._x = 15;
y = 10;
}
public Point(int x,int y) {
this._x = 15;
this.y = 10;
}
public Point(int x)
{
this._x = x;
this.y = 10;
}
public void DrawPoint() =>
Console.WriteLine($"左边点为 x: {_x}, y: {y}");
public double GetDistances(Point p) =>
Math.Pow(_x - p._x, 2) + Math.Pow(y - p.y, 2);
}
}
}
常量const、只读read-only 与只写write-only
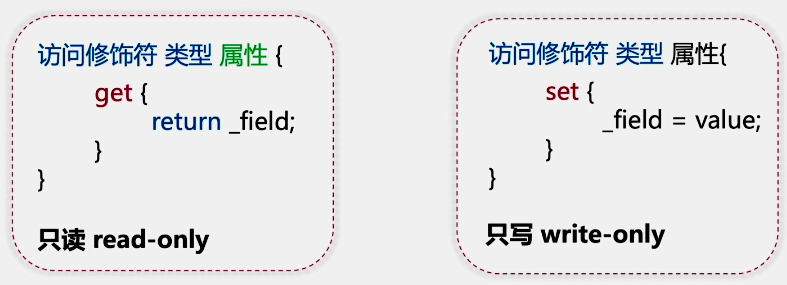
3维坐标系统拓展为5维坐标系统
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
using System.Text;
using System.Threading.Tasks;
//字段、属性与对象封装
namespace _15FieldPropertyAndObjectEncapsulation
{
internal class Program
{
static void Main(string[] args)
{
//x:15,y:10
Point a = new Point(15,10);
a.DrawPoint();
Point b = new Point(22,120);
double resule = a.GetDistances(b);
Console.WriteLine(resule);
// public, private, protected, internal
Point point = new Point(25, 30);
point.X = 30;
Console.WriteLine(point.X);
Console.Read();
return;
}
public class Point {
// 成员变量x轴,使用属性
private int _x;
public int X {
get { return this._x; }
set {
if(value < 0)
{
throw new Exception("value不能小于0");
}
this._x = value;
}
}
// 成员变量y轴,使用setY getY方法
private int y;
public void SetY(int value)
{
if (value < 0)
{
throw new Exception("value不能小于0");
}
this.y = value;
}
public int GetY() => this.y;
// 成员变量z轴,自动生成getter、setter
public int Z { get; set; }
//常量const、只读read-only 与只写write-only-------------------------------------------------------------
//时间维度,readonly
public int T { get; }
//黑洞奇点,writeonly
private int _s;
public int S { set { _s = value; } }
//第六维,常量
public const int a = 99;
//第七维,readonly常量
public readonly int b;
public Point()
{
this._x = 15;
y = 10;
T = 20;
b = 1000;
}
public Point(int x,int y) {
this._x = 15;
this.y = 10;
b = 99;
}
//常量const、只读read-only 与只写write-only-------------------------------------------------------------
public Point(int x)
{
this._x = x;
this.y = 10;
}
public void DrawPoint() =>
Console.WriteLine($"左边点为 x: {_x}, y: {y}");
public double GetDistances(Point p) =>
Math.Pow(_x - p._x, 2) + Math.Pow(y - p.y, 2);
}
}
}
索引(Index)和范围(Range)
类索引(Index)的语法结构
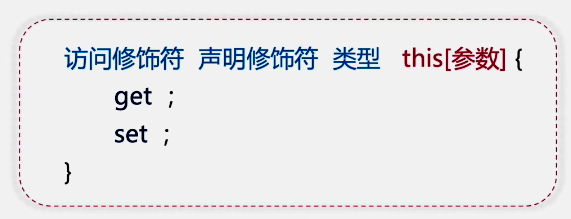
- 创建索引需要使用this关键词。
- 索引可以用于快速访问一组数据的某一项。
- 索引的使用需要通过方括号。
- 索引不能使用static、ref和out来修饰。
- 索引可以被重载。
实例
using System;
using System.Reflection;
using System.Text;
namespace HelloWorld
{
class Program
{
// 主方法
static void Main(string[] args)
{
// 2d 坐标点:{x: 15, y: 10}
//dynamic var
Point a = new Point(15, 10);
a.DrawPoint();
Point b = new Point(22, 10);
double result = a.GetDistances(b);
Console.WriteLine(result);
// public, private, protected, internal
Point point = new Point(25, 50);
point.X = 30;
//point.T = 14;
//Console.WriteLine(point.S);
//point.SetX(-30);
Console.WriteLine(point.X);
point.X = 15;
//point.a = 40;
// index 索引
string[] words = new string[]
{
// index from start index from end
"The", // 0 ^9
"quick", // 1 ^8
"brown", // 2 ^7
"fox", // 3 ^6
"jumped", // 4 ^5
"over", // 5 ^4
"the", // 6 ^3
"lazy", // 7 ^2
"dog" // 8 ^1
};
Index k = ^1;
Console.WriteLine(words[1]);
Console.WriteLine(words[k]);
Console.WriteLine(words[^2]);
Console.WriteLine(words[^3]);
Console.WriteLine(words[^4]);
// range 范围,[开始位置..结束位置]
Range p = 0..3;
var list = words[p];
for (int i = 0; i <= list.Length - 1; i++)
{
Console.WriteLine(list[i]);
}
// point 索引
Console.WriteLine(point[0]); // gamma[0], "the"
point[0] = "hello";
Console.WriteLine(point[0]);
Console.WriteLine(point["hello"]);
Console.Read();
return;
}
public class Point
{
// 成员变量x轴,使用属性
private int _x;
public int X
{
get { return this._x; }
set
{
if (value < 0)
{
throw new Exception("value不能小于0");
}
this._x = value;
}
}
// 成员变量y轴,使用setY getY方法
private int y;
public void SetY(int value)
{
if (value < 0)
{
throw new Exception("value不能小于0");
}
this.y = value;
}
public int GetY() => this.y;
// 成员变量z轴,自动生成getter、setter
public int Z { get; set; }
// 时间维度,readonly
public int T { get; }
// 黑洞奇点,wirteonly
private int _s;
public int S { set { _s = value; } }
// 第六维,常量
public const int a = 99;
// 第七维,readonly 变量
public readonly int b;
// 第八维,字符串数组
private string[] _g = new string[]
{
// index from start index from end
"The", // 0 ^9
"quick", // 1 ^8
"brown", // 2 ^7
"fox", // 3 ^6
"jumped", // 4 ^5
"over", // 5 ^4
"the", // 6 ^3
"lazy", // 7 ^2
"dog" // 8 ^1
}; // 9 (or words.Length) ^0
//类索引(Index)的语法结构-----------------------------
public string this[int index]
{
get
{
return _g[index];
}
set
{
_g[index] = value;
}
}
public int this[string str]
{
get
{
return Array.IndexOf(_g, str);
}
}
//类索引(Index)的语法结构-----------------------------
public Point()
{
this._x = 15;
y = 10;
T = 20;
//a = 40;
b = 1000;
}
public Point(int x, int y)
{
this._x = x;
this.y = y;
b = 99;
}
public Point(int x)
{
this._x = x;
this.y = 10;
}
public void DrawPoint()
{
Console.WriteLine($"左边点为 x: {_x}, y: {y}");
}
public double GetDistances(Point p) =>
Math.Pow(_x - p.X, 2) + Math.Pow(y - p.y, 2);
}
}
}
Partial Class局部类
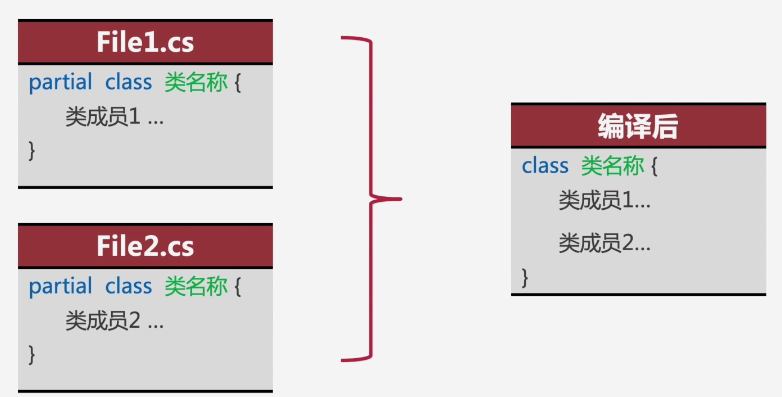
Partial 关键词
- 把一个类的内容,拆到多个文件中去,对于大型类而言可以方便地理清逻辑,还能方便多人协作。
- 子类需要有个”Partial 关键词“。
局部类的适用范围
- 类型特别大,不适合放在一个文件中实现
- 自动生成的代码,不宜与我们自己编写的代码混合在一起
- 一个类同时需要多个人同时编写的时候
注意事项
- 只适用于类、接口、结构,不支持委托和枚举
- 必须有修饰符partial
- 必须同时编译
- 必须位于相同的命名空间中
- 各部分的访问修饰符必须一致
- 具有累加效应
实例
Program.cs(主方法)
using System;
using System.Text;
namespace HelloWorld
{
class Program
{
// 主方法
static void Main(string[] args)
{
// 2d 坐标点:{x: 15, y: 10}
//dynamic var
Point a = new Point(15, 10);
a.DrawPoint();
Point b = new Point(22, 10);
double result = a.GetDistances(b);
Console.WriteLine(result);
// public, private, protected, internal
Point point = new Point(25, 50);
point.X = 30;
//point.T = 14;
//Console.WriteLine(point.S);
//point.SetX(-30);
Console.WriteLine(point.X);
point.X = 15;
//point.a = 40;
point[0] = "hello";
Console.WriteLine(point[0]); // "The"
Console.WriteLine(point["brown"]); // 2
point.D = 1;
point.printDelta();
Console.Read();
return;
}
}
}
Point.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace HelloWorld
{
//Partial 关键词
public partial class Point
{
// 成员变量x轴,使用属性
private int _x;
public int X
{
get { return this._x; }
set
{
if (value < 0)
{
throw new Exception("value不能小于0");
}
this._x = value;
}
}
// 成员变量y轴,使用setY getY方法
private int y;
public void SetY(int value)
{
if (value < 0)
{
throw new Exception("value不能小于0");
}
this.y = value;
}
public int GetY() => this.y;
// 成员变量z轴,自动生成getter、setter
public int Z { get; set; }
// 时间维度,readonly
public int T { get; }
// 黑洞奇点,wirteonly
private int _s;
public int S { set { _s = value; } }
// 第六维,常量
public const int a = 99;
// 第七维,readonly 变量
public readonly int b;
// 第八维,字符串数组
private string[] _g = new string[]
{
// index from start index from end
"The", // 0 ^9
"quick", // 1 ^8
"brown", // 2 ^7
"fox", // 3 ^6
"jumped", // 4 ^5
"over", // 5 ^4
"the", // 6 ^3
"lazy", // 7 ^2
"dog" // 8 ^1
}; // 9 (or words.Length) ^0
public string this[int index]
{
get
{
return _g[index];
}
set
{
_g[index] = value;
}
}
public int this[string name]
{
get
{
return Array.IndexOf(_g, name);
}
}
public Point()
{
this._x = 15;
y = 10;
T = 20;
//a = 40;
b = 1000;
}
public Point(int x, int y)
{
this._x = x;
this.y = y;
b = 99;
}
public Point(int x)
{
this._x = x;
this.y = 10;
}
public void DrawPoint()
{
Console.WriteLine($"左边点为 x: {_x}, y: {y}");
}
public double GetDistances(Point p) =>
Math.Pow(_x - p.X, 2) + Math.Pow(y - p.y, 2);
}
}
Point.Delta.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace HelloWorld
{
//Partial 关键词
public partial class Point
{
//第九维 delta
public int D { get; set; }
public void printDelta()
{
Console.WriteLine("我是第九维");
}
}
}
Comments | NOTHING